Developer Guide
Table of Contents
- Table of Contents
- Introduction to PetPal
- About the Developer Guide
- Setting up & Getting Started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix: Requirements
- Appendix: Instructions for manual testing
- Acknowledgements
Introduction to PetPal
PetPal is a desktop application meant for Pet Daycare owners to manage their pet clients. It is optimized for typing using the Command Line Interface (CLI) while also having a Graphical User Interface (GUI) to complement its use.
The use of PetPal can be scaled to include pet shelters, groomers or trainers.
PetPal uses Java 11 and can be run on most operating systems that support Java (e.g. Windows, macOS, Linux)
About the Developer Guide
Objectives
This guide is targeted towards potential developers that would want to contribute to the open-source project - PetPal.
This developer guide serves as a way for them to hopefully be able to learn about the overall design architecture of PetPal and the current and proposed features, detailing the design considerations and how it is implemented/going to be implemented.
The Design section makes use of various UML diagrams which were created using PlantUML
Instructions for use
General formatting conventions
- Text in blue are hyperlinks that direct you to the relevant section of the page or other websites.
- Text in bold is used to emphasize important details to look out for or to distinguish headers from the rest of the text.
- Text in
code snippets such as this
is used to show inputs and their format.
-
Note: Information that is useful to note.
-
Caution: Important information to note.
Setting up & Getting Started
Refer to the guide Setting up and getting started.
After setting up, double-click the jar file to launch PetPal.
Getting Familiar With Your User Interface
- Pet Cards: Contain all the information of a pet. (Highlighted in red)
- Command Line: Type in your commands here. (Highlighted in yellow)
- Result Display: The result of your command execution appears here. (Highlighted in blue)
- Help Button: Provides the URL of this user guide.
Design

.puml
files used to create diagrams in this document can be found in the
diagrams folder.
Refer to the PlantUML Tutorial at se-edu/guides
to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of the main components and how they interact with each other.
Main components of the architecture
Main
has two classes called Main
and MainApp
. It is responsible for,
- At app launch: Initializes the components in the correct sequence, and connect them up with each other.
- At shutdown: Shuts down the components and invoke cleanup methods where necessary.
Commons
represents a collection of classes used by multiple other components.
The rest of the App consists of four components.
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point).
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside components from being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details about each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, petListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFX UI framework. The layout of these UI parts is defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displays apet
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
How the Logic
component works:
- When
Logic
is called upon to execute a command, it uses thePetPalParser
class to parse the user command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,AddCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to add a pet). - The result of the command execution is encapsulated as a
CommandResult
object which is returned fromLogic
.
The Sequence Diagram below illustrates the interactions within the Logic
component for the execute("delete 1")
API call.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of the diagram.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
PetPalParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create anXYZCommand
object (e.g.,AddCommand
) which thePetPalParser
returns as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the pet pal data i.e., all
pet
objects (which are contained in aUniquepetList
object). - stores the currently ‘selected’
pet
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<pet>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)

Tag
list in the PetPal
, which pet
references. This allows PetPal
to only require one Tag
object per unique tag, instead of each pet
needing their own Tag
objects.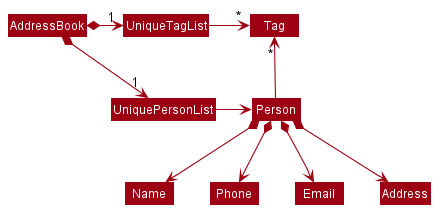
Storage component
API : Storage.java
The Storage
component,
- can save both
PetPal
data and user preference data in JSON format, and read them back into corresponding objects. - inherits from both
PetPalStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.PetPal.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.
Remind Feature
Current Implementation
The remind
mechanism is facilitated by the Deadline
and RemindCommand
, classes.
The Deadline
class has a deadline
field which is of type LocalDateTime
. Additionally, it also has a description of type String
.
‘RemindCommand’ extends from the abstract class Command
. It overrides the Command#execute()
method to filter the pet list to show only pets with Deadline
that are within 3 days from today’s date.
Given below is an example usage scenario and how the set file mechanism behaves at each step.
Given below is an example usage scenario and how the remind
mechanism behaves at each step.
Step 1. The user launches the application for the first time.
Step 2. The user decides to add a pet to the pet list. The user executes add o/Alice n/Doggo p/98765432 e/example@gmail.com a/311, Clementi Ave 2, #02-25 ts/2023-03-27 21:09:09 d/Feed dog - 2023-03-27 21:09:09 t/Dog t/Chihuahua
command to add a pet named Doggo
with a reminder to feed the dog and deadline of 2023-03-27 21:09:09
to the pet list. The add
command calls the AddCommand#execute()
method.
Step 3. The user may exit and reopen PetPal at a future date. The user intends to see all deadlines that are due soon. The user executes the remind
command to filter the pet list to show only pets with Deadline
that are within 3 days from today’s date. The remind
command calls the RemindCommand#execute()
method.
Step 4. The RemindCommand#execute()
method calls the Model#updateFilteredPetList()
method to filter the pet list to show only pets with Deadline
that are within 3 days from today’s date.
The following sequence diagram shows how the remind
operation works:
The following activity diagram summarizes what happens when a user executes a new command:
Design Considerations:
Choice 1 (Current Choice): Filter pet list upon command
- Pros:
- User can easily find upcoming deadlines.
- Cons:
- Counterintuitive since reminders shouldn’t need user input to be shown.
Choice 2: Alert users of upcoming deadlines upon startup
- Pros:
- User will be reminded of upcoming deadlines upon startup.
- Cons:
- Might be annoying to users who don’t want to be reminded of upcoming deadlines.
Calculator Feature
Current Implementation
The calculator mechanism is facilitated by the AddCommand
class.
The calculator calculates the amount of money owed by pet owners to the daycare owners,
based on an initial timestamp of type java LocalDateTime
that is required to be non-null when
entering the Add
command.
Given below is an example usage scenario and how the calculator mechanism behaves at each step:
Step 1. The user launches the application for the first time.
Step 2. The user decides to add a pet to the pet list. The user executes
add o/Alice n/Doggo p/98765432 e/example@gmail.com a/311, Clementi Ave 2, #02-25 ts/2023-03-27 21:09:09 d/Feed dog -
2023-03-27 21:09:09 t/Dog t/Chihuahua
command to add a pet named Doggo
a with reminder to feed the dog and the deadline of
2023-03-27 21:09:09
to the pet list. The add
command calls the AddCommand#execute()
method.
Step 3. The user may exit and reopen PetPal at a future date. The user will see the amount due to them as a field in each pet card by their respective owners.
Step 4. The amount updates upon clicking on the PetCard on the panel, or upon restarting the client.
The calculator feature is not an additional command and does not have an activity or sequence diagram.
Archive Feature
Current Implementation
The archive mechanism is facilitated by the ArchiveCommand
class.
The ArchiveCommand#execute()
adds the provided Pet
into an archive list and deletes the Pet
from the pet list,
the Pet
must exist in the pet list, and the index provided must be a valid index of a pet in the current viewable pet list
Given below is an example usage scenario and how the set file mechanism behaves at each step:
Step 1. The user launches the application for the first time
Step 2. The user decides to archive a pet in the pet list. The user executes `archive 1`
Step 3. The user can view the archived pets in the data/archive.json file
Extensions:
Step 2a. The PetPal list does not have any pets, the pet at list position 1 does not exist
3a. The PetPal returns an error message: `The provided index is out of bounds`
The following sequence diagram shows how the archive operation works:
The following activity diagram summarizes what happens when a user executes a new archive command:
Highlight Feature
Current Implementation
The highlight mechanism is facilitated by the ‘PetListPanel’, ‘Pet’, and ‘MarkCommand’ classes. This feature highlights pets that have not been marked and have a deadline within a day in the GUI. The highlight feature will be executed automatically every certain time window without user input to support a real-time state.
Given below is an example usage scenario and how the highlight mechanism behaves at each step:
Step 1. The user launches the application.
Step 2. The user decides to add two pets to the pet list with a deadline due three days later.
Step 3. The given pet will not be highlighted at this moment.
Step 4. The user decides to mark the first pet as done.
Step 5. The user exit the application and decided to reopen it two days later.
Step 6. The second pet that has not been marked will be highlighted while the first pet will not be highlighted since it was already marked.
The following activity diagram summarizes what happens during the process:
Design Considerations:
Aspect: How to reduce human error:
Alternative 1 (Current Choice): Automatically execute the feature after every certain period
- Pros:
- Shows real-time state.
- Will not show outdated list state.
- Cons:
- Uses more memory to execute the feature at every time.
Alternative 2: Provide a Refresh button to update the pet list
- Pros:
- Use less memory since it will be executed only when needed.
- Cons:
- User might forget to refresh to the updated state and shows the outdated instead.
Undo Feature
Current Implementation
The undo mechanism is facilitated by the ModelManager
and UndoCommand
, classes.
The ModelManager
class is implemented by PetPal and has a petPalCache
field which is of typePetPal
.
‘UndoCommand’ extends from the abstract class Command
. It overrides the Command#execute()
method to filter the pet list to show only pets with Deadline
that are within 3 days from today’s date.
Given below is an example usage scenario and how the undo mechanism behaves at each step:
Step 1. The user launches the application for the first time. Step 2. The user decides to add a pet to the pet list. The user executes `add o/Alice n/Doggo p/98765432 e/example@gmail.com a/311, Clementi Ave 2, #02-25 ts/2023-03-27 21:09:09 d/Feed dog - 2023-03-27 21:09:09 t/Dog t/Chihuahua` command to add a pet named `Doggo` with a reminder to feed the dog and deadline of `2023-03-27 21:09:09` to the pet list. The `add` command calls the `AddCommand#execute()` method. Step 3. The user realises that he has made a mistake and executes `undo`. Step 4. The list displayed returns to the previous state without the new Doggo added.
Extensions:
Step 2a. The user decides to delete a pet from the pet list. The user executes `delete 1`. 2b. The user realises that he has made a mistake and executes `undo`. 2c. The list displayed returns to the previous state with item 1 which was just deleted.
The following sequence diagram shows how the undo
operation works:
The following activity diagram summarizes what happens when a user executes a new command:
Design considerations:
-
Current implementation : Before any command, a cache of the previous state of the archive and PetPal is saved
into a PetPal object. Undo returns the
Archive
andModel
to this previously saved state.- Pros: Easier to implement
- Cons: Might be memory inefficient
[Proposed] Add medical key information to pet (not shown in UI)
Proposed Implementation
The proposed function is an extension of the base PetPal
, uses a Medical
class to store medical information, and users will be able to input medical information.
Design considerations:
-
Alternative 1 (current choice) : Store information such as vaccination information in the Medical Class
Users will only be able to access it if they type in a keyword such as “vaccination” or “vaccine” followed by their password
which they will have to set at the start of the application.
- Pros: Provides a way for users to store medical information without having to worry about it being shown in the UI
- Cons: Might be hard for users to remember the password
-
Alternative 2 : Show all the information in the UI
- Pros: Easier and more intuitive for users to use
- Cons: Not secure, anyone can see the information
[Proposed] Importing data from Excel (CSV)
Proposed Implementation
The proposed importing function is an extension of the base PetPal
and uses a CsvToJsonParser
to convert CSV data
to application readable JSON data.
Design considerations:
-
Alternative 1 (current choice): Write an external script that parses the CSV data based on the column names
into a JSON save file that works with PetPal, which they will then put into the data file before starting PetPal
for PetPal to be able to read and modify the imported data
- Pros: Might be easier to implement
- Cons: Might be confusing for users to use (running an external script)
-
Alternative 2: Provide an interface for users to upload their CSV data into PetPal and automatically parse
the data into JSON format and refreshes the database.
- Pros: Easier and more intuitive for users to use
- Cons: Builds upon Alternative 1, requiring more work to implement
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile:
- needs to manage a significant number of pet details
- prefer desktop apps over other types
- can type fast
- prefers typing to mouse interactions
- is reasonably comfortable using CLI apps
- prefers an app with GUI.
Value proposition: manage pet details better than a typical mouse-driven app
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
new staff | see usage instructions | refer to instructions when I forget how to use the app |
* * * |
staff | add a new pet | |
* * * |
staff | input pet details | track the details of each pet |
* * * |
staff | delete a pet | remove entries that I no longer need |
* * * |
staff | find a pet by name | locate details of pets without having to go through the entire list |
* * * |
staff | tag pets | take note of pet personalities or special requirements to know their needs |
* * |
forgetful pet owner | get a reminder for my pet | remember to fetch my pets from the daycare |
* * |
pet owner | keep track of my own pets | know that my pets are taken care of well |
* * |
staff | keep track of pet locations | account for missing pets |
* * |
forgetful staff | get a reminder for pets | track pets that have overstayed their duration |
* * |
staff | input pet attendance | track pet attendance in the daycare |
* * |
staff | view pet appointment dates | bring pets to vet if necessary |
* * |
staff | search for a pet via tags | cater different services to different pets |
* * |
staff | schedule appointment dates | remember when pet owners are coming to pick up or drop off their pets |
* * |
staff | look at vaccination status of pets | know what vaccinations required are missing |
* * |
staff | archive pet information | keep a record of older pet clients |
* * |
friendly staff | send personal messages to pet owners | update or help pet owners |
* * |
general staff | write and save notes for pets | track additional information if needed |
* * |
staff | export pet data | backup pet data |
* * |
new staff | import pet data | |
* |
staff | input feedback for pet owners | let the pet owners know how to better care for their pets’ behaviours |
* |
pet owner | give staff feedback | let the staff know how to improve their services |
* |
pet owner | check pet attendance | track how long my pet has been in daycare |
* |
pet owner | look at staff comments on the feedback | acknowledge staff feedback and give clarification |
* |
business owner | have an overview on the pet welfare | know that pet owners and staffs are satisfied |
* |
staff | receive pet owner feedback | know how to take better care of pets |
* |
pet owner | view daycare availability | check if there are slots available to board my pet |
* |
pet owner | view facilities | know the available amenities for my pets |
* |
clueless pet owner | look for pet daycare locations | know which facilities are available |
* |
poor staff | keep track of hours worked | keep track of earnings |
* |
business owner | keep track of money owed by pet owners |
Use cases
(For all use cases below, the System is PetPal
and the Actor is the user
, unless specified otherwise)
Use case: View help
- Actor requests to view help
- System shows help message
Use case ends.
Use case: List the list of pets
MSS
- Actor requests to list pets
- System displays list
Use case ends.
Extensions
- 2a. There are no pet details.
Use case ends.
Use case: Add a pet
MSS
- Actor requests to add a pet
- System deletes the pet
Use case ends.
Extensions
- 2a. Any required detail(s) are missing
- 2a1. System shows an error message.
Use case ends.
- 2a1. System shows an error message.
Use case: View reminders
MSS
- Actor requests to see reminders that are due soon
- System filters the pet list to show desired pets
Use case ends.
Use case: Find pet by name
MSS
- Actor requests to find pet(s) by name(s)
- System filter the list to show the desired pet(s)
Use case ends.
Use case: Edit a pet
Use case: View Cost of Pet
MSS
- Actor requests to change a pet’s cost calculation rate and additional flat cost
- System updates cost based on calculation
- Update is done when the user clicks on another PetCard
Use case: View Cost of Pet
MSS
- Actor requests to change a pet’s cost calculation rate and additional flat cost
- System edits the pet’s cost calculation formula
Use case ends.
Extensions
- 2a. Any required detail(s) are missing
- 2a1. System shows an error message.
Use case ends.
- 2a1. System shows an error message.
Use case: Mark deadline
MSS
- Actor requests to list pets
- System shows a list of pets
- Actor requests to mark deadline for a specific pet
- System marks the pet
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends. -
3a. The given index is invalid.
- 3a1. System shows an error message.
Use case resumes at step 2.
- 3a1. System shows an error message.
Use case: Delete a pet
MSS
- Actor requests to list pets
- System shows a list of pets
- Actor requests to delete a specific pet from the list
- System deletes the pet
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends. -
3a. The given index is invalid.
- 3a1. System shows an error message.
Use case resumes at step 2.
- 3a1. System shows an error message.
Use case: Archive a pet
MSS
- Actor requests to list all pets
- System shows the list of all pets
- Actor requests to archive a specific pet in the list
- System archives the pet
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends. - 3a. The given index is invalid.
- 3a1. System shows an error message.
Use case resumes at step 2.
- 3a1. System shows an error message.
- 3b. The given pet exists in the archive
- 3b1. System shows an error message.
Use case resumes at step 2.
- 3b1. System shows an error message.
Use case: Clear the pet list
MSS
- Actor requests to list all pets
- System shows the list of all pets
- Actor requests to clear the list
- System clears the list
Use case: Undo a command
MSS
- Actor requests a
add
,delete
orarchive
- System executes
- Actor realises he made a mistake and
undo
- System returns to the previous state
Use case: Exit the System
MSS
- Actor requests to exit
- System exits
Use case ends.
Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
11
or above installed. - Should be able to hold up to 500 pets without a noticeable sluggishness in performance for typical usage.
- A user with above-average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Glossary
| Term | Definition | |—————|—————————————————————————————————-| | Mainstream OS | Refers to Windows, Linux, Unix, MacOS | | JSON | The data format used to store PetPal saves, consisting of a name/value pair | | CSV | Stands for Comma-seperated-values, a type of file where information/values are seperated by commas |
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy it into an empty folder
-
Double-click the jar file.
Expected: Shows the GUI with a set of sample contacts
-
-
Saving window preferences
-
Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location are retained.
-
Deleting a pet
-
Deleting a pet while all pets are being shown
-
Prerequisites: List all pets using the
list
orl
command. Multiple pets in the list. -
Test case:
delete 1
Expected: First Pet entry is deleted from the list. Details of the deleted pet entry are shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No pet is deleted. Error details are shown in the status message. The status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is an integer larger than the list size),delete abc
, (where abc is a string or special characters)
Expected: Similar to previous.
-
-
{ more test cases … }
Saving data
-
Viewing the save files
- Exit the app by typing
exit
ore
.
Expected: A new folder calleddata
is created in the folder where your jar file is at - Double-click the
data
folder.
Expected: 2 files,petpal.json
(which includes sample data) andarchive.json
present in thedata
folder
- Exit the app by typing
-
Dealing with missing data files
- To simulate a missing data file, go to the
data
folder and deletepetpal.json
and/orarchive.json
- Restart PetPal and type
exit
- The missing files are re-created in the
data
folder with the sample data populated.
-
Note:
archive.json
does not contain any pet entries by default
- To simulate a missing data file, go to the
-
Dealing with corrupted data files
- To simulate a corrupted data file, go to the
data
folder and edit thepetpal.json
and/orarchive.json
with random strings not of JSON format - Start PetPal, the corrupted data file is replaced with the sample data
- The corrupted files are re-created in the
data
folder with sample data
-
Note:
archive.json
does not contain any pet entries by default
- To simulate a corrupted data file, go to the
Acknowledgements
- This project is based on the AddressBook-Level3 project created by the SE-EDU initiative
- Libraries used: JavaFX, Jackson, JUnit5